Introduction¶
This tool is used in three phases.
- First we define a circuit containing multiple devices.we connect those and maybe define some callback functions.
- In the second phase we simulate the circuit, define plots of interest.
- And in the third phase we optimize the circuit.
A simple example¶
First we define a simple circuit
We use two amplifiers in series
A Low Noise Amp and a Driver
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | import rf_linkbudget as rf
import matplotlib.pyplot as plt
import numpy as np
cr = rf.Circuit('SimpleEx')
lna = rf.Amplifier("LNA TQL9066",
Gain=[(0, 18.2)],
NF=0.7,
OP1dB=21.5,
OIP3=40)
drv = rf.Amplifier("Driver TQP3M9028",
Gain=[(0, 14.9)],
NF=1.7,
OP1dB=21.4,
OIP3=40)
src = rf.Source("Source")
sink = rf.Sink("Sink")
|
Now we have to define connections between the devices
1 2 3 | src['out'] >> lna['in']
lna['out'] >> drv['in']
drv['out'] >> sink['in']
|
that we can simulate the circuit correctly, we have to define where the signal is applied to.
A Port as example.
We do this by using a callback function.
First we define it with an inline function, then connect this function to the Port by using the memberfunction called regCallback.
1 2 3 4 5 6 | # create callback function
def cb_src(self, f, p):
return {'f': f, 'p': p, 'Tn': rf.RFMath.T0}
src['out'].regCallback(cb_src) # connect callback to Port
|
after defining all the callbacks we can finalize the circuit
1 | cr.finalise()
|
and simulate the circuit
1 2 3 4 5 6 7 8 9 | sim = cr.simulate(network=cr.net,
start=cr['Source'],
end=cr['Sink'],
freq=[100e6],
power=np.arange(-50, -10, 1.0))
h = sim.plot_chain(['p'])
plt.show()
|
We see that we have to define a start port and an end port.
In this case its intelligent enough to choose the cr[‘Source’][‘out’] port and the cr[‘Sink’][‘in’] port.
Also we have to define the frequency and input power range of the simulation
(Source code, png, hires.png, pdf)
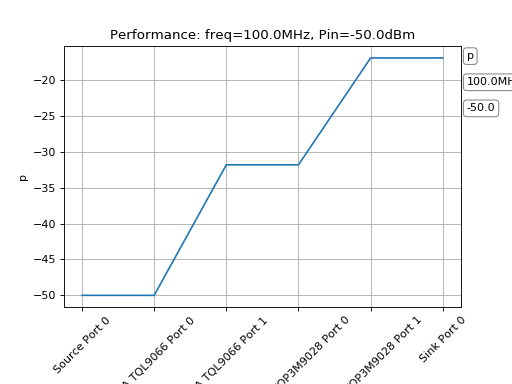
And here we see our output!
In this case we see the signal power p from the source to the sink.
We can also show other parameters like noisefigure, signal-to-noise ratio, spurious-free-dynamic-range and others.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 | import rf_linkbudget as rf
import matplotlib.pyplot as plt
import numpy as np
cr = rf.Circuit('SimpleEx')
lna = rf.Amplifier("LNA TQL9066",
Gain=[(0, 18.2)],
NF=0.7,
OP1dB=21.5,
OIP3=40)
drv = rf.Amplifier("Driver TQP3M9028",
Gain=[(0, 14.9)],
NF=1.7,
OP1dB=21.4,
OIP3=40)
src = rf.Source("Source")
sink = rf.Sink("Sink")
src['out'] >> lna['in']
lna['out'] >> drv['in']
drv['out'] >> sink['in']
# create callback function
def cb_src(self, f, p):
return {'f': f, 'p': p, 'Tn': rf.RFMath.T0}
src['out'].regCallback(cb_src) # connect callback to Port
cr.finalise()
sim = cr.simulate(network=cr.net,
start=cr['Source'],
end=cr['Sink'],
freq=[100e6],
power=np.arange(-50, -10, 1.0))
h = sim.plot_chain(['p'])
plt.show()
|